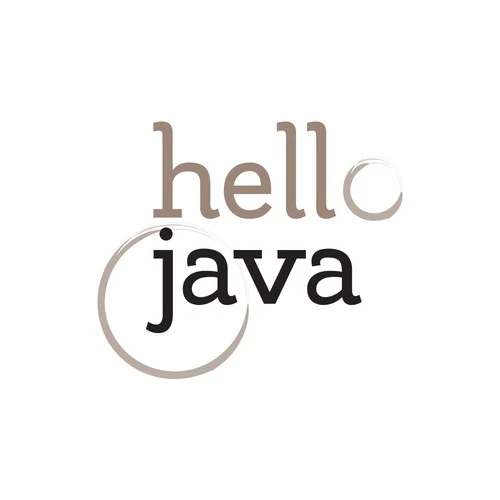
Review Exercises
Solutions Manual: Chapter 2 Big Java, by Cay Horstmann 1. Review Exercises.
R2.1. An object contains state information. An object reference describes the
location of an object. R2.3. An object is a value that can be created, stored and
manipulated. A class is a programmer defined data type that describes objects
with the ...
Part of the document
Review Exercises
R2.1
An object contains state information. An object reference describes the
location of an object.
R2.3
An object is a value that can be created, stored and manipulated. A class
is a programmer defined data type that describes objects with the same
behavior.
R2.5
new BankAccount(5000) is an object of class BankAccount, whereas
BankAccount momsSavings; declares a variable of class BankAccount. You can
assign an object to a variable that has the same type, for example:
BankAccount momsSavings = new BankAccount(5000);
R2.7
A local variable is declared and initialized in the body of a method. A
parameter variable is declared in themethod header and initialized when the
method is called.
R2.9
The first statement does not initialize the variable b. The second
statement initializes the variable to an object reference that refers to a
newly constructed bank account object.
R2.11
new Rectangle(75, 75, 50, 50);
new Greeter("Mars");
new BankAccount(5000);
R2.13
. Rectangle r =(5,10,15,20);
. Missing new Rectangle
. double x = BankAccount(10000).getBalance();
. Missing new
. BankAccount b;
. b.deposit(10000);
.
. b not initialized.
. b = new BankAccount(10000); b.add("one million bucks");
. The BankAccount class doesn't have a method called add
R2.15
BankAccount b = new BankAccount(10);
. b has the value 10
b.deposit(5000);
. b now has value 5010
b.withdraw(b.getBalance() / 2);
. b now has value 2505
R2.17
The this reference is used to refer to the implicit parameter of a method..
Programming Exercises
P2.1
ExP2_1.java
import java.awt.Rectangle;
/**
Displays a rectangle and then translates it 3 times.
*/
public class ExP2_1
{
public static void main(String[] args)
{
Rectangle r1 = new Rectangle(0, 0, 5, 7);
System.out.println(r1);
r1.translate(5, 0);
System.out.println(r1);
r1.translate(0, 7);
System.out.println(r1);
r1.translate(-5, 0);
System.out.println(r1);
}
}
P2.3
Greeter.java
/**
A class whose objects emit personalized greetings.
*/
public class Greeter
{
/**
Constructs a greeter.
@param aName the name of the person to be greeted
*/
public Greeter(String aName)
{
name = aName;
}
/**
Makes a "Hello" greeting.
@return the greeting
*/
public String sayHello()
{
String message = "Hello, " + name + "!";
return message;
}
/**
Makes a "Goodbye" greeting.
@return the greeting
*/
public String sayGoodbye()
{
String message = "Goodbye, " + name + "!";
return message;
}
private String name;
}
ExP2_3.java
/**
Tests the greeter that can say goodbye.
*/
public class ExP2_3
{
public static void main(String[] args)
{
Greeter g = new Greeter("John");
Greeter h = new Greeter("Mary");
System.out.println(g.sayHello());
System.out.println(h.sayHello());
System.out.println(h.sayGoodbye());
System.out.println(g.sayGoodbye());
}
}
}
P2.5
BankAccount.java
/**
A bank account has a balance that can be changed by
deposits and withdrawals.
*/
public class BankAccount
{
/**
Constructs a bank account with a zero balance
*/
public BankAccount()
{
balance = 0;
}
/**
Constructs a bank account with a given balance
@param initialBalance the initial balance
*/
public BankAccount(double initialBalance)
{
balance = initialBalance;
}
/**
Deposits money into the bank account.
@param amount the amount to deposit
*/
public void deposit(double amount)
{
double newBalance = balance + amount;
balance = newBalance;
}
/**
Withdraws money from the bank account.
@param amount the amount to withdraw
*/
public void withdraw(double amount)
{
double newBalance = balance - amount;
balance = newBalance;
}
/**
Gets the current balance of the bank account.
@return the current balance
*/
public double getBalance()
{
return balance;
}
private double balance;
}
ExP2_5.java
/**
Tests the bank account class.
*/
public class ExP2_5
{
public static void main(String[] args)
{
BankAccount account = new BankAccount();
account.deposit(1000);
account.withdraw(500);
account.withdraw(400);
double balance = account.getBalance();
System.out.println("The balance is $" + balance);
}
}
P2.7
SavingsAccount.java
/**
A savings account has a balance that can be changed by
deposits and withdrawals.
*/
public class SavingsAccount
{
/**
Constructs a savings account with a zero balance
*/
public SavingsAccount()
{
balance = 0;
interestRate = 0;
}
/**
Constructs a savings account with a given balance
@param initialBalance the initial balance
@param rate the interest rate in percent
*/
public SavingsAccount(double initialBalance,
double rate)
{
balance = initialBalance;
interestRate = rate;
}
/**
Deposits money into the savings account.
@param amount the amount to deposit
*/
public void deposit(double amount)
{
double newBalance = balance + amount;
balance = newBalance;
}
/**
Withdraws money from the savings account.
@param amount the amount to withdraw
*/
public void withdraw(double amount)
{
double newBalance = balance - amount;
balance = newBalance;
}
/**
Gets the current balance of the savings account.
@return the current balance
*/
public double getBalance()
{
return balance;
}
/**
Adds interest to the savings account.
*/
public void addInterest()
{
double interest = balance * interestRate / 100;
balance = balance + interest;
}
private double balance;
private double interestRate;
}
ExP2_7.java
/**
Tests the savings account class.
*/
public class ExP2_7
{
public static void main(String[] args)
{
SavingsAccount momsSavings
= new SavingsAccount(1000, 10);
momsSavings.addInterest();
momsSavings.addInterest();
momsSavings.addInterest();
momsSavings.addInterest();
momsSavings.addInterest();
double balance = momsSavings.getBalance();
System.out.println("The balance is $" + balance);
}
}
P2.9
Employee.java
/**
An employee with a name and salary.
*/
public class Employee
{
/**
Constructs a default employee with a blank name
and a salary of $0.
*/
public Employee()
{
name = "";
salary = 0;
}
/**
Constructs an employee.
@param n the employee name
@param s the employee salary
*/
public Employee(String n, double s)
{
name = n;
salary = s;
}
/**
Gets the employee name.
@return the name
*/
public String getName()
{
return name;
}
/**
Gets the employee salary.
@return the salary
*/
public double getSalary()
{
return salary;
}
/**
Raises the salary by a given percentage.
@param percent the percentage of the raise
*/
public void raiseSalary(double percent)
{
salary = salary + (salary / percent);
}
private String name;
private double salary;
}
ExP2_9.java
/**
This program tests the Employee class.
*/
public class ExP2_9
{
public static void main(String[] args)
{
Employee employee = new Employee("Harry Hacker", 50000);
System.out.println(employee.getName() + "'s salary is: $"
+ employee.getSalary());
employee.raiseSalary(10);
System.out.println("After the raise, " + employee.getName()
+ "'s salary is: $" + employee.getSalary());
}
}
P2.11
Student.java
/**
A student who is taking quizzes.
*/
public class Student
{
/**
Constructs a default student with no name.
*/
Student()
{
name = "";
totalScore = 0;
quizCount = 0;
}
/**
Constructs a student with a given name.
@param n the name
*/
Student(String n)
{
name = n;
totalScore = 0;
quizCount = 0;
}
/**
Gets the name of this student.
@return the name
*/
public String getName()
{
return name;
}
/**
Adds a quiz score.
@param the score to add
*/
public void addQuiz(int score)
{
totalScore = totalScore + score;
quizCount = quizCount + 1;
}
/**
Gets the sum of all quiz scores.
@return the total score
*/
public double getTotalScore()
{
return totalScore;
}
/**
Gets the average of all quiz scores.
@return the average score
*/
public double getAverageScore()
{
return totalScore / quizCount;
}
private String name;
private double totalScore;
private int quizCount;
}
ExP2_11.java
/**
This program tests the Student class.
*/
public class ExP2_11
{
public static void main(String[] args)
{
Student student = new Student("Cracker, Carl");
student.addQuiz(8);
student.addQuiz(6);
student.addQuiz(9);
System.out.println(student.getName() + "'s quiz score total is "
+ student.getTotalScore() + ", and the average is "
+ student.getAverageScore());
}
}
P2.13
Circle.java
/**
A circle with a given radius.
*/
public class Circle
{
/**
Constructs a circle with radius 0.
*/
Circle()
{
radius = 0;
}
/**
Constructs a circle with a given radius.
@param r the radius
*/
Circle(double r)
{
radius = r;
}
/**
Gets the area of the circle.
@return the area
*/
public double getArea()
{
return Math.PI * radius * radius;
}
/**
Gets the perimeter of the cir