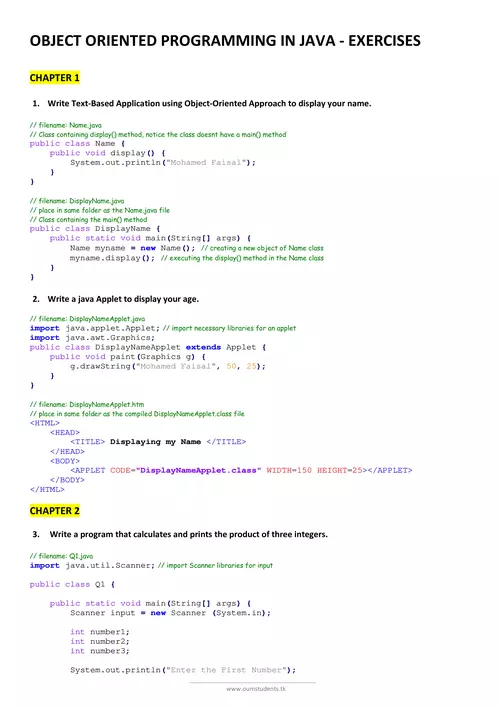
OBJECT ORIENTED PROGRAMMING IN JAVA - EXERCISES
JDK that makes it possible to run Java programs independently of whether or class contains special support that allows literal strings and string-valued.
Part of the document
www.oumstudents.tk
OBJECTORIENTEDPROGRAMMINGINJAVAͲEXERCISES
CHAPTER1
1. WriteTextͲBasedApplicationusingObjectͲOrientedApproachtodisplayyourname.
// filename: Name.java
// Class containing display() method, notice the class doesnt have a main() method public class Name {
public void display() {
System.out.println("Mohamed Faisal");
}
}
// filename: DisplayName.java
// place in same folder as the Name.java file
// Class containing the main() method
public class DisplayName { public static void main(String[] args) {
Name myname = new Name(); // creating a new object of Name class
myname.display(); // executing the display() method in the Name class
}
}
2. WriteajavaApplettodisplayyourage.
// filename: DisplayNameApplet.java
import java.applet.Applet; // import necessary libraries for an applet
import java.awt.Graphics;
public class DisplayNameApplet extends Applet { public void paint(Graphics g) {
g.drawString("Mohamed Faisal", 50, 25);
}
}
// filename: DisplayNameApplet.htm
// place in same folder as the compiled DisplayNameApplet.class file
Displaying my Name
CHAPTER2
3. Writeaprogramthatcalculatesandprintstheproductofthreeintegers.
// filename: Q1.java
import java.util.Scanner; // import Scanner libraries for input
public class Q1 {
public static void main(String[] args) { Scanner input = new Scanner (System.in);
int number1;
int number2;
int number3;
System.out.println("Enter the First Number");
www.oumstudents.tk
number1 = input.nextInt();
System.out.println("Enter the Second Number");
number2 = input.nextInt();
System.out.println("Enter the Third Number");
number3 = input.nextInt();
System.out.printf("The product of three number is %d:", number1 * number2 *
number3);
}
}