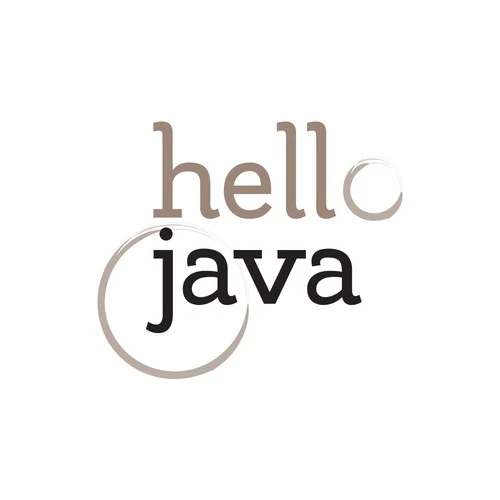
Alice - Temple CIS
[B HD] Copying an Instruction. [B HD] Testing (and Debugging) a Generic Method
. [A HD] Chapter Summary. [A HD] Review Questions. [A HD] Exercises .... This
approach to programming will serve you well in any language you use ? Java,
Visual Basic, C, C++, Python ? and is one of the driving principles behind much
of ...
Part of the document
Links to Chapter 2 Sections ...
[A HD] Top-Down Design and Modular Development 4
[A HD] A Program Development Cycle 11
[B HD] Desiging Methods 11
[B HD] Coding Methods in Alice 12
[B HD] Testing and Debugging Alice Methods 13
[A HD] Tutorial 2A - Working with Primitive Methods in Alice 16
[B HD] Running Primitive Methods Directly 19
[B HD] Using Primitive Camera Methods to Find a
Lost Object 21
[A HD] Tutorial 2B - Creating New Methods in Alice 23
[B HD] Setting the Scene 24
[B HD] Designing a Method 28
[B HD] Coding the Jump Methods 29
[B HD] Creating the Main Method 32
[B HD] Testing (and Debugging) Your Finished
Software 33
[BT] Tutorial 2C - Generic Methods and Parameters 34
[B HD] Designing a Generic Method 35
[B HD] Creating a Parameter 37
[B HD] Coding a Generic Instruction 38
[B HD] Copying an Instruction 39
[B HD] Testing (and Debugging) a Generic Method
40
[A HD] Chapter Summary 41
[A HD] Review Questions 44
[A HD] Exercises Error! Bookmark not defined.
Alice - An Introduction to Programming Using Virtual Reality
Last edited June 28th,2005 by Charles Herbert. Edited by Frank Friedman,
August 21st 2005
Chapter 2 - Designing Methods in Alice
Goal of this lesson:
By the end of this lesson students should have a basic understanding of how
to design and implement methods in Alice.
Learning objectives for this lesson:
After finishing this chapter, you should be able to:
1. Provide brief definitions of the following terms: CamelCase,
coding, encapsulated methods, integration test, method header,
modular development, off-camera, parameter, primitive methods,
program development cycle, reusable code, test for correctness,
testing shell, top-down design, unit test, user-defined methods,
variable.
2. Describe the processes known as top-down design and modular
development, including why they are often used together, how
organizational charts are involved, and some of the advantages of
modular development of new software.
3. List and describe the steps in a simple program development cycle.
4. Describe the difference between primitive methods and user-created
methods in Alice, and show how to run primitive methods to directly
to position objects and manipulate the camera in an Alice world.
5. Create new methods in Alice in a manner that demonstrates good top-
down design and modular development.
6. Create and demonstrate the use of generic methods in Alice that
contain method parameters.
Top-Down Design and Modular Development
The process of developing methods for objects is mostly a process of
developing algorithms, since each method is an algorithm. Algorithm
development has a long history in the world of mathematics, where
algorithms are step-by-step solutions to well-defined mathematical
problems, such as finding the least common denominator of two fractions.
Traditional approaches to mathematical problem solving have been applied to
many different disciplines that rely on mathematics, such as engineering,
the physical and social sciences, and computer programming. Generally, a
problem must be clearly specified so that once a solution has been
developed it can be compared to the specifications to determine its
correctness.
It's easier to solve smaller problems than it is to solve big
ones, so good mathematicians often start solving a problem by trying
to break a large algorithm into parts that are more manageable. This
process of decomposition is sometimes called a divide and conquer
approach to problem solving, in which a problem is broken into parts,
those parts are solved individually, then the smaller solutions are
assembled into a big solution.
Top-Down or Step-Wise Development
Computer programmers do the same thing when developing new software.
This process is also known as top-down or stepwise development,
starting at the top with one concept or big idea, and then breaking
that down into several parts. If those parts can be further broken
down, then the process continues. The end result is a collection of
small solutions, called modules, which collectively contain the
overall solution to the original problem.
Let's look at an example of top-down development. Imagine that
you want to write a computer program to process the payroll for a
large company. Your big idea is named "Payroll". What would you need
to do if you were going to process a payroll? Generally, you would
need to get some data, perform some calculations, and output some
results. Figure 2-2 shows Payroll broken down into these three
modules.
[pic]
Figure 2-1. a payroll program broken down into three modules.
You can go further in decomposing the problem. There are two
different sets of data - old data, such as a list of employees, and
new data, such as the number of hours each person worked during a
specific week. The old data will probably be read in from a data file
on the hard disk drive, while the new data will be entered into the
system for the first time, so the Get Data module can be broken down
further, into Get Old Data and Get New Data. If the Calculations and
Output Results modules are broken down in a similar manner, and then
the sub-modules of those modules are broken down, and so on, then the
final design might look something like the one in Figure 2-2. Such a
diagram is called an organizational chart.
[pic]
Figure 2-2. An organizational chart showing a payroll program composed of
many modules.
Organizational charts show the overall structure of separate
units that have been organized to form a single complex entity. The
chart shows how the upper levels are broken down into the parts at the
lower levels, and conversely, how the parts at the lower levels are
layered together to form the upper levels. Organizational charts are
most commonly seen describing hierarchies of organization, such as
government agencies. In this case, you can see how the specific
modules further down the chart are combined to form the overall
payroll program. A programmer, or a team of programmers working
together, can create each of the modules as software methods, and then
put them together to build the entire payroll program.
The process of top-down design leads to modular development, in
which the parts, or modules, that make up a complete solution to a
problem are developed individually and then combined to form that
complete solution. Modular development of computer software has the
following advantages, especially for larger projects:
1. Modular development makes a large project more manageable. Smaller
and less complex tasks are easier to understand than larger ones and
are less demanding of resources.
2. Modular development is faster for large projects. You can have
different people work on different modules, and then put their work
together. This means that different modules can be developed at the
same time, which speeds up the overall project.
3. Modular development leads to a higher quality product. Programmers
with knowledge and skills in a specific area, such as graphics,
accounting, or data communications, can be assigned to the parts of
the project that require those skills.
4. Modular development makes it easier to find and correct errors in
computer programs. Often, the hardest part of correcting am error in
computer software is finding out exactly what is causing the error.
Modular development makes it easier to isolate the part of the
software that is causing trouble.
5. Most importantly, modular development increases the reusability of
your solutions. Solutions to smaller problems are more likely to be
useful elsewhere than solutions to bigger problems.
Reusable Code
This last point is one of the most important concepts in all of
computer programming. Saving solutions to small problems so they can
be applied elsewhere creates reusable code. In fact, most of the
software on a computer system is filled with layers of reusable code,
since computer programs contain many small tasks that need to be
repeated, such as getting a user's name and password before running a
program. Everything from the low-level parts of the operating system
that directly control the hardware, to the most complex user
applications is filled with layers of short programming modules that
are constantly re-used in different situations.
Reusable code makes programming easier because you only need to
develop the solution to a problem once, then you can call up that code
whenever you need it. You can also save the modules you have
developed as part of one software development project, and then reuse
them later as parts of other projects, modifying them if necessary to
fit new situations. Over time, you can build libraries of software
modules for different tasks.
Object-oriented programming, by its very nature, encourages such
development of reusable code. Methods to perform simple tasks can be
reused within an object to form more complicated methods, and entire