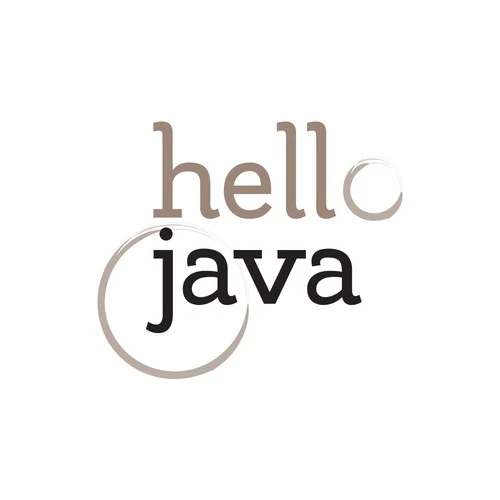
laboratory exercises - DMU
CSCI1402 Introductory Java Programming Array Introduction Exercises.
CSCI1402 Introductory Java Programming. Week 5 Laboratory - Introduction to
arrays.
Part of the document
CSCI1402 Introductory Java Programming
Week 5 Laboratory - Introduction to arrays
NOTICE:
You must keep a FOLDER OF LABORATORY WORK (PORTFOLIO) that will include
both 'paper and pen' exercises and computer printouts of your programs. The
code should be well commented and the printouts should be annotated by
hand. After testing your programs, copy the program output, paste it under
your code and enclose it in comment symbols.
Each program must have a header with
- your name,
- course,
- date
- exercise ID (academic week and the exercise number)
- a brief description of the program.
'Pen and paper ' exercises - work out the answers yourself and write them
down on paper. After finishing several exercises on paper enter the code
into the computer, compile and run the program.
Compare the program output with your results.
Exercise 1 (Pen & paper)
a) Declare and create an array called
- moreNumbers, of size 100, containing type short
- prices, of size 11, containing type double
- characters, of size 50, containing type char
- names, of size 80, containing type String
- shoppingList, of size 5, containing type String
b) Complete the table below with a name of each array and the relevant
values
array name first index last index
moreNumbers 0 99
prices .
characters
names
shoppingList
c) Write down the required expressions:
the 1st element of the array shoppingList is
the 2nd element of the array shoppingList is
the 5th element of the array shoppingList is
d) Use the array attribute length to find the length of the array
moreNumbers
e) Use the length attribute to express the index of the last element
of the array prices
f) For the array shoppingList answer the following
- assign the values "milk", "eggs", "bread", "butter", "cheese" into the
elements of the array
- display the value in the 3rd element of the array
- assign value "cake" into the last element of the array
- find the length of the array
Exercise 2
a) Create a new Java program called ArrayProgram in your CSCI1402 folder
- declare and create an array newNumbers which can contain 12 int
numbers
- assign these values into newNumbers
10, 6, 88, 91, 25, 77, 14, 23 ,4, 235, 66, 81.
- find and display the length of the array
- display the value stored in the 5th element of the array
-change the value in the 5th element to 35. Display again.
- calculate and display the average of the values stored in the array
(use the length attribute)
Exercise 3
Extend your program ArrayProgram to
- declare and create an array to hold the module code CSCI1401 as a list of
char
values. Draw the resulting array.
- Change the value in the last element to 2 and output the array contents.
Exercise 4
Write down your answers, then add the code to your ArrayProgram:
What is the output of the following code segments?
a)
int[] array = {8,6,9,7,6,4,4,5,8,10};
System.out.println("Index Value");
for (int index =0; index