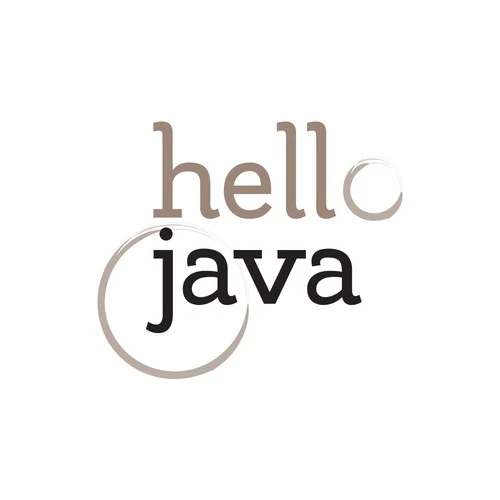
If-else exercises:
Write a Java program which reads a year (integer) from the user and decides
whether ... Challenge 1: If you finish the exercises early, try to solve this problem.
Part of the document
If-else exercises:
1. Redo the pounds to kilogram program written before which was:
Our program will convert a weight expressed in pounds into kilograms.
a. Our input is the weight in pounds.
b. Our output is the weight in kilograms
c. We also know that Kilograms = Pounds / 2.2
. Let's take another look at the program which converts pounds to
kilograms.
. Since a weight cannot be a negative number, our program should not
accept a negative number as a valid weight.
. Let's rewrite the program to print an error message if the weight
entered in pounds is negative.
2. An Auto Insurance Program
. Example - Write a program to determine the cost of an automobile
insurance premium, based on driver's age and the number of accidents
that the driver has had.
. The basic insurance charge is $500. There is a surcharge of
$100 if the driver is under 25 and an additional surcharge for
accidents:
# of accidents Accident Surcharge
1 50
2 125
3 225
4 375
5 575
6 or more No insurance
3. Prompt the user for a number and print good if the number is greater
than 5, between 8 & 10 or greater than 33. Otherwise, print bad. Use the ||
operator in your if statement.
4. Prompt the user for two words. If they are the same, print Great - the
same. If they are the same except for the case, print Okay - almost the
same. If they are the same length, print At least the same length. You
will need the following string functions:
. compareTo(otherString) (which returns true if matched)
. compareToIgnoreCase(otherString) (which returns true if matched
regardless of case)
. length() (which returns the length)
5. Write a program that recommends the number of calories a person should
eat each day. Calories are units of energy found in all foods. Base your
recommendation on the person's weight and whether the person has an active
or sedentary(inactive) lifestyle. If the person is sedentary, that person's
activity factor is 13. If the person is active, that person's activity
factor is 15. Multiply the activity factor by the person's weight to get
the recommended number of calories. Start your program by:
. having the user enter their weight, as a floating point number;
. having the user enter whether they have active or sedentary lifestyle,
as a character, 'A' for active or 'S' sedentary;
. use a switch (or else if) selection statement to use the appropriate
calculation for the recommended calories for the selected lifestyle;
. print out your results on the screen.
6. Write a Java program which reads a year (integer) from the user and
decides whether that year is a leap year. A year is a leap year (and so
contains a February 29) if it is divisible by 4. But if the year is also
divisible by 100 then it is not a leap year, unless it is divisible by
400. This means that years such as 1992, 1996 are leap years because they
are divisible by 4 and are not affected by the rest of the rule which
applies to century years such as 1900 and 2000. Century years are not leap
years except where they are a multiple of 400. Hence, the years 1700, 1800
and 1900 were not leap years and did not contain a February 29. But the
year 2000 was a leap year, the first such century leap year since 1600.
Challenge 1: If you finish the exercises early, try to solve this
problem. Write a Java program that reads a date from the user in
numeric form. For example, February 17, 2003 would be entered as the
three integers 2, 17, and 2003. Your program must then determine if
the date is a "valid" date. Use the following information to
determine if the date is valid: January, March, May, July, August,
October, and December all have 31 days. April, June, September, and
November all have 30 days. February has 28 days in a non-leap year
and 29 days in a leap year. Echo the input and print either "valid
date" or "invalid date" as output.
7. Extend #6 to use a window pane interface instead of a system console.
Instead of printing to and from the console window, print to and from a
JOptionPane. The format is:
String yourVariable = (JOptionPane.showInputDialog("Ask a question"));
You can only enter a string, but you can convert a string to an
Integer with:
Integer.parseInt(stringValue) and to a double with
Double.parseDouble(stringValue).
To print out:
When you test, you may find your window pops up behind the other
windows, so be sure to minimize everything.
JOptionPane.showMessageDialog(null, "Good job! sweetener is " +
value3);
Sample prompting with JOptionPane:
import javax.swing.JOptionPane;
public class mouse{
public static void main (String [] args){
String value = JOptionPane.showInputDialog("Enter Sweetner name need to
kill mouse in grams here");
JOptionPane.showMessageDialog(null, "Good job! sweetener is " + value);
int value2 = Integer.parseInt(JOptionPane.showInputDialog("Enter integer
Sweetner need to kill mouse in grams here"));
JOptionPane.showMessageDialog(null, "Good job! sweetener is " + value2);
double value3 = Double.parseDouble(JOptionPane.showInputDialog("Enter
Double Sweetner need to kill mouse in grams here"));
JOptionPane.showMessageDialog(null, "Good job! sweetener is " + value3);
}}