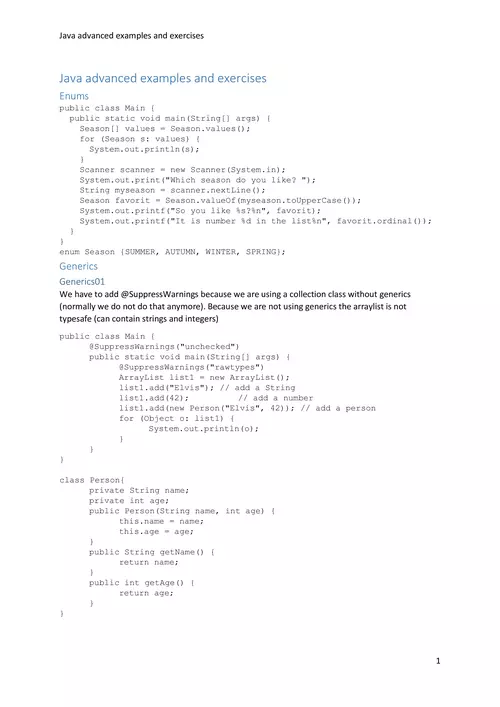
Java advanced examples and exercises - Beta vzw |
Java advanced examples and exercises public static void main(String[] args) { When we declare the arraylist as an arraylist of Strings
Part of the document
Java advanced examples and exercises
1
Java advanced examples and exercises
Enums
public class Main {
public static void main(String[] args) {
Season[] values = Season.values();
for (Season s: values) {
System.out.println(s);
}
Scanner scanner = new Scanner(System.in);
System.out.print("Which season do you like? ");
String myseason = scanner.nextLine();
Season favorit = Season.valueOf(myseason.toUpperCase());
System.out.printf("So you like %s?%n", favorit);
System.out.printf("It is number %d in the list%n", favorit.ordinal());
}
}
enum Season {SUMMER, AUTUMN, WINTER, SPRING};
Generics
Generics01
We have to add @SuppressWarnings because we are using a collection class without generics
(normally we do not do that anymore). Because we are not using generics the arraylist is not
typesafe (can contain strings and integers)
public class Main {
@SuppressWarnings("unchecked")
public static void main(String[] args) {
@SuppressWarnings("rawtypes")
ArrayList list1 = new ArrayList();
list1.add("Elvis"); // add a String
list1.add(42); // add a number
list1.add(new Person("Elvis", 42)); // add a person
for (Object o: list1) {
System.out.println(o);
}
}
}
class Person{
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Java advanced examples and exercises
2
Generics02
When we declare the arraylist as an arraylist of Strings (ArrayList) it is impossible to add
integers:
public class Main {
public static void main(String[] args) {
ArrayList list1 = new ArrayList();
list1.add("Elvis");
//list1.add(42); //not possible
//list1.add(new Person("Elvis", 42)); //not possible
for(String s:list1) {
System.out.println(s);
}
}
}
Generics03
We deciare our own generic Trap-class:
public class Trap {
private E prisoner;
public void catchPrisoner(E prisoner) {
this.prisoner = prisoner;
}