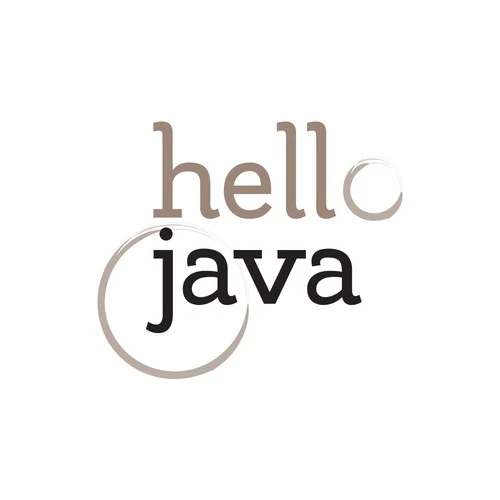
Chapter 7 Review Exercise Solutions
The bounds are the lowest and highest legal index value; that is, 0 and one less
than the length of the array/size of the array list. A bounds error occurs when an ...
import java.util.ArrayList;. public class ExR7_3. {. public static void main(String[]
args). {. ArrayList<BankAccount> accounts = new ArrayList<BankAccount>();.
Part of the document
Chapter 7 Review Exercise Solutions
R7.1
An index is an integer value which represents a position in an array or
array list.
The bounds are the lowest and highest legal index value; that is, 0 and one
less than the length of the array/size of the array list.
A bounds error occurs when an array or array list is accessed at an index
that is outside the bounds.
R7.2
public class ExR7_2
{
public static void main(String[] args)
{
String[] greeting = new String[2];
greeting[0] = "Hello,";
greeting[1] = "cruel";
greeting[2] = "World!";
}
}
When you run this program, you get an array index out of bounds exception.
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 2
at ExR7_2.main(ExR7_2.java:8)
The line number (8 in the example) helps you locate the error.
R7.3
import java.util.ArrayList;
public class ExR7_3
{
public static void main(String[] args)
{
ArrayList accounts = new ArrayList();
accounts.add(new BankAccount(1,1500));
accounts.add(new BankAccount(2,2500));
accounts.add(new BankAccount(3,1000));
accounts.add(new BankAccount(4, 500));
BankAccount max = accounts.get(0);
BankAccount min = accounts.get(0);
for (BankAccount a : accounts)
{
if (a.getBalance() > max.getBalance())
max = a;
if (a.getBalance() < min.getBalance())
min = a;
}
System.out.println(max.getAccountNumber() + " "
+ max.getBalance());
System.out.println(min.getAccountNumber() + " "
+ min.getBalance());
}
}
R7.4
import java.util.Scanner;
import java.util.ArrayList;
/**
Reads 10 strings and inserts them into an array list. Then,
prints out the strings in the opposite order from which they
were entered.
*/
public class ExR7_4
{
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
ArrayList strings = new ArrayList();
System.out.println(
"Enter ten strings, each ended by a newline.");
for (int i = 0; i < 10; i++)
strings.add(in.nextLine());
for (int i = strings.size() - 1; i >= 0; i--)
System.out.println(strings.get(i));
}
}
R7.5
for (BankAccount a : accounts)
{
if (largestYet == null)
largestYet = a;
else
if (a.getBalance() > largestYet.getBalance())
largestYet = a;
}
return largestYet;
This approach is less efficient because we perform a check at every
iteration (largestYet==null), even though we know that check is only going
to be true on the first iteration of the loop.
R7.6
The maximum value in the list could be smaller than 0 (i.e. negative).
Then, the program would incorrectly set max to 0. We can fix the error by
applying the same approach as in the function that finds the largest value
in a list (as detailed in the book). Using this approach, we set max to be
the first element of the list, and then iterate through the remaining
elements, updating max if needed.
R7.7
a. 1 2 3 4 5 6 7 8 9 10
for (int i = 0; i < 10 ; i++ )
a[i] = i + 1;
b. 0 2 4 6 8 10 12 14 16 18 20
for (int i = 0; i