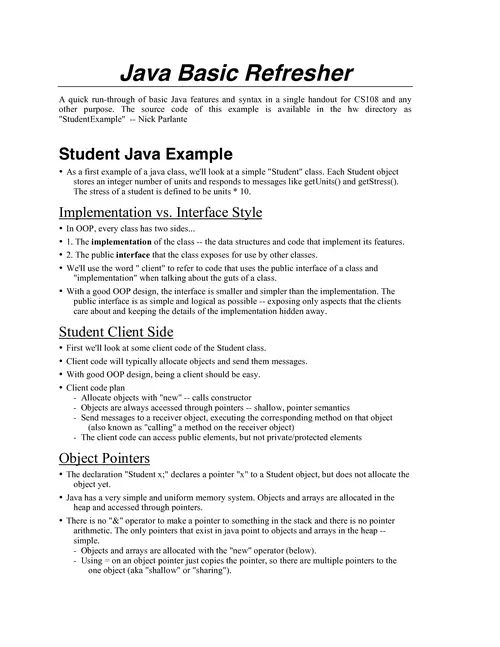
Java Basic Refresher
Coding Exercise Of The Week - Java Programming Challenges - Week 1. Learn Java - Exercise 01x - Methods in Java Java tutorial for complete.
Part of the document
Java Basic Refresher A quick run-through of basic Java features and syntax in a single handout for CS108 and any other purpose. The source code of this example is available in the hw directory as "StudentExample" -- Nick Parlante Student Java Example • As a first example of a java class, we'll look at a simple "Student" class. Each Student object stores an integer number of units and responds to messages like getUnits() and getStress(). The stress of a student is defined to be units * 10. Implementation vs. Interface Style • In OOP, every class has two sides... • 1. The implementation of the class -- the data structures and code that implement its features. • 2. The public interface that the class exposes for use by other classes. • We'll use the word " client" to refer to code that uses the public interface of a class and "implementation" when talking about the guts of a class. • With a good OOP design, the interface is smaller and simpler than the implementation. The public interface is as simple and logical as possible -- exposing only aspects that the clients care about and keeping the details of the implementation hidden away. Student Client Side • First we'll look at some client code of the Student class. • Client code will typically allocate objects and send them messages. • With good OOP design, being a client should be easy. • Client code plan - Allocate objects with "new" -- calls constructor - Objects are always accessed through pointers -- shallow, pointer semantics - Send messages to a receiver object, executing the corresponding method on that object (also known as "calling" a method on the receiver object) - The client code can access public elements, but not private/protected elements Object Pointers • The declaration "Student x;" declares a pointer "x" to a Student object, but does not allocate the object yet. • Java has a very simple and uniform memory system. Objects and arrays are allocated in the heap and accessed through pointers. • There is no "&" operator to make a pointer to something in the stack and there is no pointer arithmetic. The only pointers that exist in java point to objects and arrays in the heap -- simple. - Objects and arrays are allocated with the "new" operator (below). - Using = on an object pointer just copies the pointer, so there are multiple pointers to the one object (aka "shallow" or "sharing").