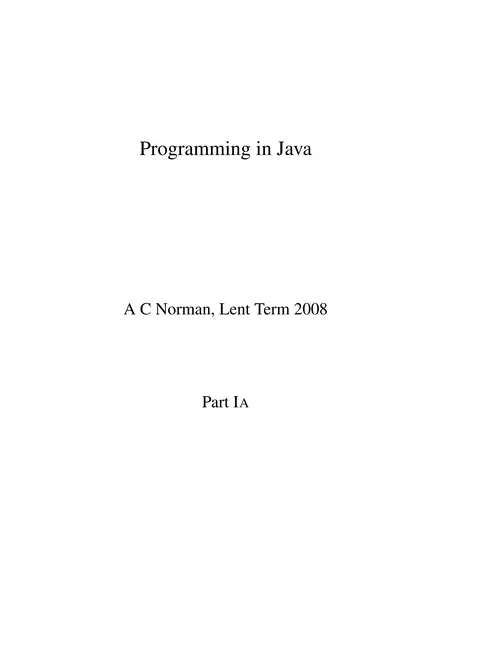
Programming in Java - Department of Computer Science and
If you ally habit such a referred java programming exercises with solutions books that will present you worth, get the very best seller from us currently
Part of the document
Programming in Java
A C Norman, Lent Term 2008
Part IA
2
Contents1 Preface7
1.1 What is programming about? . . . . . . . . . . . . . . . . . . . . 7
1.2 What aboutgoodprogramming? . . . . . . . . . . . . . . . . . . 8
1.3 Ways to save time and effort . . . . . . . . . . . . . . . . . . . . 9
1.3.1 Use existing resources . . . . . . . . . . . . . . . . . . . 9
1.3.2 Avoid dead-ends . . . . . . . . . . . . . . . . . . . . . . 10
1.3.3 Create new re-usable resources . . . . . . . . . . . . . . . 10
1.3.4 Documentation and Test trails . . . . . . . . . . . . . . . 10
1.3.5 Do not make the same mistake twice . . . . . . . . . . . . 10
1.4 Where does Java fit in? . . . . . . . . . . . . . . . . . . . . . . . 12
2 General advice for novices13
3 Introduction15
3.1 Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
3.1.1 Books . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18
3.2 Practical work . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
3.2.1 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 24
3.3 A Cook-book Kick-start . . . . . . . . . . . . . . . . . . . . . . 28
3.3.1 Code Layout . . . . . . . . . . . . . . . . . . . . . . . . 33
3.3.2 Emacs . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
3.3.3 Drawing to a window: JApplets . . . . . . . . . . . . . . 37
3.3.4 HTML and appletviewer . . . . . . . . . . . . . . . . . . 42
3.3.5 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 43
4 Basic use of Java49
4.1 Data types, constants and operations . . . . . . . . . . . . . . . .49
4.1.1 Reserved Words . . . . . . . . . . . . . . . . . . . . . . 49
4.1.2 Basic Types . . . . . . . . . . . . . . . . . . . . . . . . . 51
4.1.3 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 65
4.2 Operators and expressions . . . . . . . . . . . . . . . . . . . . . 71
3
4CONTENTS
4.2.1 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 74
4.3 Control structures . . . . . . . . . . . . . . . . . . . . . . . . . . 77
4.3.1 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 77
4.4 Control structures Part 2 . . . . . . . . . . . . . . . . . . . . . . 82
4.4.1 Expression Statements . . . . . . . . . . . . . . . . . . . 82
4.4.2 Blocks . . . . . . . . . . . . . . . . . . . . . . . . . . . 82
4.4.3 Null statements . . . . . . . . . . . . . . . . . . . . . . . 83
4.4.4if. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83
4.4.5while,continueandbreak. . . . . . . . . . . . . . 84
4.4.6do. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 84
4.4.7for. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 85
4.4.8switch,caseanddefault. . . . . . . . . . . . . . 85
4.4.9return. . . . . . . . . . . . . . . . . . . . . . . . . . 87
4.4.10try,catchandthrow,finally. . . . . . . . . . . 87
4.4.11assert. . . . . . . . . . . . . . . . . . . . . . . . . . 88
4.4.12 Variable declarations . . . . . . . . . . . . . . . . . . . . 88
4.4.13 Method definitions . . . . . . . . . . . . . . . . . . . . . 89
4.4.14 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 90
4.5 Java classes and packages . . . . . . . . . . . . . . . . . . . . . . 98
4.5.1 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 108
4.6 Inheritance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 115
4.6.1 Inheritance and the standard libraries . . . . . . . . . . . 116
4.6.2 Name-spaces and classes . . . . . . . . . . . . . . . . . . 120
4.6.3 Program development with classes . . . . . . . . . . . . . 125
4.7 Generics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 129
4.7.1 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 130
4.8 Important features of the class libraries . . . . . . . . . . . .. . . 139
4.8.1 File input and output . . . . . . . . . . . . . . . . . . . . 140
4.8.2 Big integers . . . . . . . . . . . . . . . . . . . . . . . . . 147
4.8.3 Collections . . . . . . . . . . . . . . . . . . . . . . . . . 150
4.8.4 Simple use of Threads . . . . . . . . . . . . . . . . . . . 150
4.8.5 Network access . . . . . . . . . . . . . . . . . . . . . . . 153
4.8.6 Menus, scroll bars and dialog boxes . . . . . . . . . . . . 155
4.8.7 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 160
5 Designing and testing programs in Java 167
5.1 Different sorts of programming tasks . . . . . . . . . . . . . . . .171
5.2 Analysis and description of the objective . . . . . . . . . . . .. . 179
5.2.1 Important Questions . . . . . . . . . . . . . . . . . . . . 179
5.2.2 Informal specifications . . . . . . . . . . . . . . . . . . . 180
5.2.3 Formal descriptions . . . . . . . . . . . . . . . . . . . . . 181
CONTENTS5
5.2.4 Executable specifications . . . . . . . . . . . . . . . . . . 181
5.3 Ethical Considerations . . . . . . . . . . . . . . . . . . . . . . . 182
5.4 How much of the work has been done already? . . . . . . . . . . 183
5.5 What skills and knowledge are available? . . . . . . . . . . . . . 185
5.6 Design of methods to achieve a goal . . . . . . . . . . . . . . . . 186
5.6.1 Top-Down Design . . . . . . . . . . . . . . . . . . . . . 186
5.6.2 Bottom-Up Implementation . . . . . . . . . . . . . . . . 189
5.6.3 Data Centred Programming . . . . . . . . . . . . . . . . 190
5.6.4 Iterative Refinement . . . . . . . . . . . . . . . . . . . . 190
5.6.5 Which of the above is best? . . . . . . . . . . . . . . . . 191
5.7 How do we know it will work? . . . . . . . . . . . . . . . . . . . 191
5.8 While you are writing the program . . . . . . . . . . . . . . . . . 194
5.9 Documenting a program or project . . . . . . . . . . . . . . . . . 195
5.10 How do we know it does work? . . . . . . . . . . . . . . . . . . . 197
5.11 Is it efficient? . . . . . . . . . . . . . . . . . . . . . . . . . . . . 200
5.12 Identifying errors . . . . . . . . . . . . . . . . . . . . . . . . . . 201
5.13 Corrections and other changes . . . . . . . . . . . . . . . . . . . 204
5.14 Portability of software . . . . . . . . . . . . . . . . . . . . . . . 205
5.15 Team-work . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 206
5.16 Lessons learned . . . . . . . . . . . . . . . . . . . . . . . . . . . 207
5.17 Final Words . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 208
5.18 Challenging exercises . . . . . . . . . . . . . . . . . . . . . . . . 208
6 A representative application219
6.1 A Lisp interpreter . . . . . . . . . . . . . . . . . . . . . . . . . . 219
6.1.1 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . 233
7 What you do NOT know yet235
8 Model Examination Questions237
8.1 Java vs ML . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 237
8.2 Matrix Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 238
8.3 Hash Tables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 238
8.4 Compass Rose . . . . . . . . . . . . . . . . . . . . . . . . . . . . 239
8.5 Language Words . . . . . . . . . . . . . . . . . . . . . . . . . . 239
8.6 Exception abuse . . . . . . . . . . . . . . . . . . . . . . . . . . . 240
8.7 Queues . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 240
8.8 Loops . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 240
8.9 Snap . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 240
8.10 Partitions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 241
8.11 Laziness . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 241
6CONTENTS
8.12 Cryptarithmetic . . . . . . . . . . . . . . . . . . . . . . . . . . . 242
8.13 Bandits . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 242
8.14 Exception . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 244
8.15 Features . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 245
8.16 More features . . . . . . . . . . . . . . . . . . . . . . . . . . . . 245
8.17 Debate . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 246
8.18 Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 246
8.19 Filter (Coffee?) . . . . . . . . . . . . . . . . . . . . . . . . . . . 246
8.20 Parse trees . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 247
8.21 Big Addition . . . . . . . . . . . . . . . . . . . . . . . . . . . . 248
8.22 Lists in Java . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 248
8.23 Pound, Shillings and Ounces . . . . . . . . . . . . . . . . . . . . 248
8.24 Details . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 249
8.25 Name visibility . . . . . . . . . . . . . . . . . . . . . . . . . . . 250
8.26 Several Small Tasks . . . . . . . . . . . . . . . . . . . . . . . . . 250
8.27 Some Tiny Questions . . . . . . . . . . . . . . . . . . . . . . . . 251
9 Java 1.5 or 5.0 versus previous versions 253
9.1 An enhancedforloop . . . . . . . . . . . . . . . . . . . . . . . 253
9.2 Generics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 254
9.3assert. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 254
9.4 Static imports . . . . . . . . . . . . . . . . . . . . . . . . . . . . 254
9.5 Auto-boxing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 254
9.6 Enumerations . . . . . . . . . . . . . . . . . . . . . . . . . . . . 254
9.7printf. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 255
9.8 Scanner . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 255
9.9 Variable numbers of arguments for methods . . . . . . . . . . . .255
9.10 Annotations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 256
9.11 Enhanced concurrency control . . . . . . . . . . . . . . . . . . . 256
Chapter 1Preface1.1 What is programming about?There are two stories you can tell yourself about what this course is going to do for
you. The first is the traditional one that it is so you can learnsome Java. Acquire
knowledge and skills. The second, which may be more interesting, is to see this
course as part of your journey as you start to become (or at least appreciate what
it is to be) a Computer Scientist. This second perspective suggests that there may
be something for you here whether or not you believe you are already skilled in
Java, and it challenges you to look beyond the mere details tothe tought patterns
that link them together.
In the early days of computers programming involved a full understanding of
the way that the hardware of your computer worked, your program, when run,
took over essentially the whole machine and it had to includeeverything needed
to manage input and output. In extreme cases one started the process of load-
ing code into a computer by using hand-switches to place bit-patterns directly
into the machine's memory. After a while operating systems came along and
provided serious insulation from that level of extreme awareness of hardware, and
high-level languages make it possible to express programs in at least semi-human-
understandable form. But still the emphasis was on "writing aprogram", which
tended to be a stand-alone application that solved some problem.
Libraries of pre-written sub-programs grew up, but for a very long time the
ones that anybody could rely on having access to were either rather specialist or
the functionality that they provided was at a rather low and boring level. There
were libraries that could really help you with serious tasks(such as building a
windowed user-interface) but none of them gained really global acceptance, and
only a few were of any use on more than one brand of computer. The libraries that
were standard with typical programming languages providedfor fairly limited file