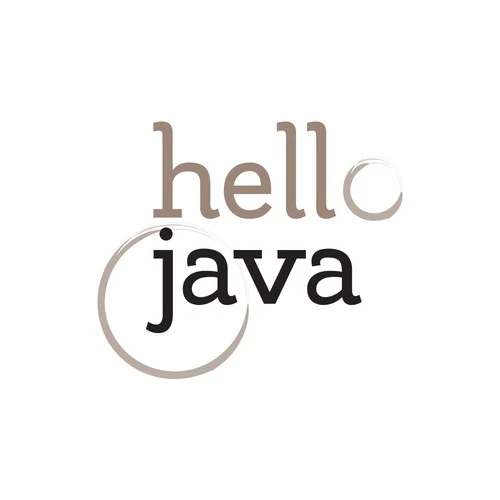
Lab 8 ? Exceptions - UNL CSE
Activities/Exercises. Exception handling using the throws clause and a try-catch
block. Propagating exceptions. Working with the finally clause. Modifying a
program with multiple catch blocks. Activity 1: Exception handling using the
throws clause and a try-catch block. Download TestExceptions.java from the
course website.
Part of the document
Lab 8 - Exceptions
Student Handout
1. Lab Objectives
Following the lab you should be able to:
. Define what an exception is and why exceptions occur.
. Explain the advantages of using Java exception handling over
traditional error management techniques.
. Distinguish between checked and unchecked exceptions.
. Write a simple exception-handling routine to handle a single exception
using try-catch and try-finally blocks.
. Write methods that use the throws statement for exception handling.
2. Prior to the laboratory
. Review the laboratory handout.
. Read Wu, Chapter 8.
. Read Chapters 80-81,
http://chortle.ccsu.ctstateu.edu/cs151/cs151java.html
. Read the tutorial, "Handling Errors with Exceptions,"
http://java.sun.com/docs/books/tutorial/essential/exceptions/index.html
|Topics Covered in Lab |
|Basics of compile time and runtime errors |
|What is an exception? |
|Why must exceptions be handled? |
|Advantages of Java exception handling |
|Exception Types: |
|Checked versus Unchecked |
|Arithmetic exceptions, IO exceptions, pointer exceptions, and indexing |
|exceptions |
|Exception handling |
|The try block |
|The catch block(s) |
|The finally block |
|Throwing exceptions |
|The throws statement |
|Handling multiple exceptions |
3. Topics
4. Activities/Exercises
1. Exception handling using the throws clause and a try-catch block.
2. Propagating exceptions.
3. Working with the finally clause.
4. Modifying a program with multiple catch blocks.
Activity 1: Exception handling using the throws clause and a try-catch
block
. Download TestExceptions.java from the course website.
. Compile TestExceptions.java.
. Notice the error message in the bottom JGrasp panel; you will see the
unreported exceptions and which line of code is responsible for this
exception.
. Complete Question 1 on your worksheet for Activity 1.
. Modify the main() method declaration in TestExceptions.java to propagate
the IOException using a throws clause.
. Compile the modified version of TestExceptions.java.
. Why does the program now compile when you only handled one of the
exceptions listed?
. Hint: Look at FileNotFoundException in the Java API.
. Complete Question 2a on your worksheet for Activity 1.
. Execute the program and notice the message in the output window. Because
there is no file: Sample.jpg, your main() method throws the exception.
. Modify TestExceptions.java to handle the IOException using a try-catch
block instead of propagating the exception. When the exception occurs,
print the following string:
"The file you have requested cannot be found."
. After you have made your changes, compile and execute
TestExceptions.java. You should see your error message in the bottom
JGrasp panel instead of the thrown exception.
. Complete Question 2b on your worksheet for Activity 1.
. Complete Question 3 on your worksheet for Activity 1.
Activity 2: Propagating exceptions
. Download CallStack.java from the course website.
. Study the CallStack.java code to determine what it does.
. Compile and execute CallStack.java.
. Complete Question 1 (a and b) on your worksheet for Activity 2.
. Modify the main() method to handle the exception propagated up
the call chain to it. Use a try-catch block to display a meaningful
error message when the exception occurs.
. Compile and run the program.
. Complete Question 2 on your worksheet for Activity 2.
. Notice that although the exception was thrown in func2, it is
caught by the catch block in the main method.
Activity 3: Working with the finally clause
. Download TestFinally.java and the text file 'trycatch.txt' from
the class website into your lab 8 directory.
. Study the TestFinally.java code to determine what it is supposed
to do.
. Compile TestFinally.java.
. Complete Question 1 on your worksheet for Activity 3.
. Modify TestFinally.java to handle the exception by adding a try-
catch block to the main() method. Follow the instructions (numbered 1
- 5) in the comments of the file.
. The first line of the catch block should look like this:
catch(IOException ex) {.
. Display a meaningful error message to indicate the error has
occurred. Also include the following Java statement in your catch
clause (this assumes you use 'ex' as your variable name in the catch
clause): System.out.println("The exception is: " + ex);
. You should have two System.out statements in your catch clause.
. Adding the finally clause: Even when an error occurs in a
method, there may be clean-up activities that are required before the
method or program terminates. The finally clause will execute if the
all the statements in the try block execute (and nothing in a catch
block) or if an exception is thrown and the statements in one of the
catch blocks are executed.
. In this case, any open files should be closed prior to leaving the
method and program termination.
. Modify TestFinally.java to include a finally block. The code in the
finally block should close the file that was successfully opened and
print a message indicating the file is now closed. HINT: You will need
to also add a try-catch block inside the finally block to handle any
IOExceptions associated with the file close operation.
. Compile and execute your modified version of TestFinally.java to
test that it works.
. You should now see that your code has generated another type of
exception.
. Complete Question 2a and 2b on your worksheet for Activity 3.
. Add a second catch clause inside the finally block with a
meaningful message to handle this new exception.
. Compile and execute your modified version of TestFinally.java to
test that it works.
Activity 4: Modifying a program with multiple catch blocks
. Download MultiCatch.java from the class website.
. Study the MultiCatch.java code to determine what it does.
. Compile the program MultiCatch.java.
. Execute MultiCatch with no arguments:
java MultiCatch
. Complete Question 1 on your worksheet for Activity 4.
. Running a program with arguments in JGrasp: From the Run menu in your
CSD window, click on the box in front of Run Arguments. This will
open a TextField labeled: Run Arguments right above your code in the
CSD window. This is where you will enter the arguments: abc xyz in the
following instruction.
. Execute MultiCatch with two arguments:
java MultiCatch abc xyz
. Complete Questions 2 and 3 on your worksheet for Activity 4.
5. Supplemental Resources
Exception Handling in Java, http://www.churchillobjects.com/c/11012.html
Exceptions in Java, http://www.javaworld.com/javaworld/jw-07-1998/jw-07-
exceptions.html
6. Think About
. What types of errors or exceptions might be generated when dealing
with files?
. Can you guarantee that the program you have written handles all
possible exceptions?
. Why do we have two types of exceptions: checked and unchecked?
. When should you use a finally block?
. If you do not catch and handle exceptions, what will happen if the
program throws an exception?